Polymorphism to Java
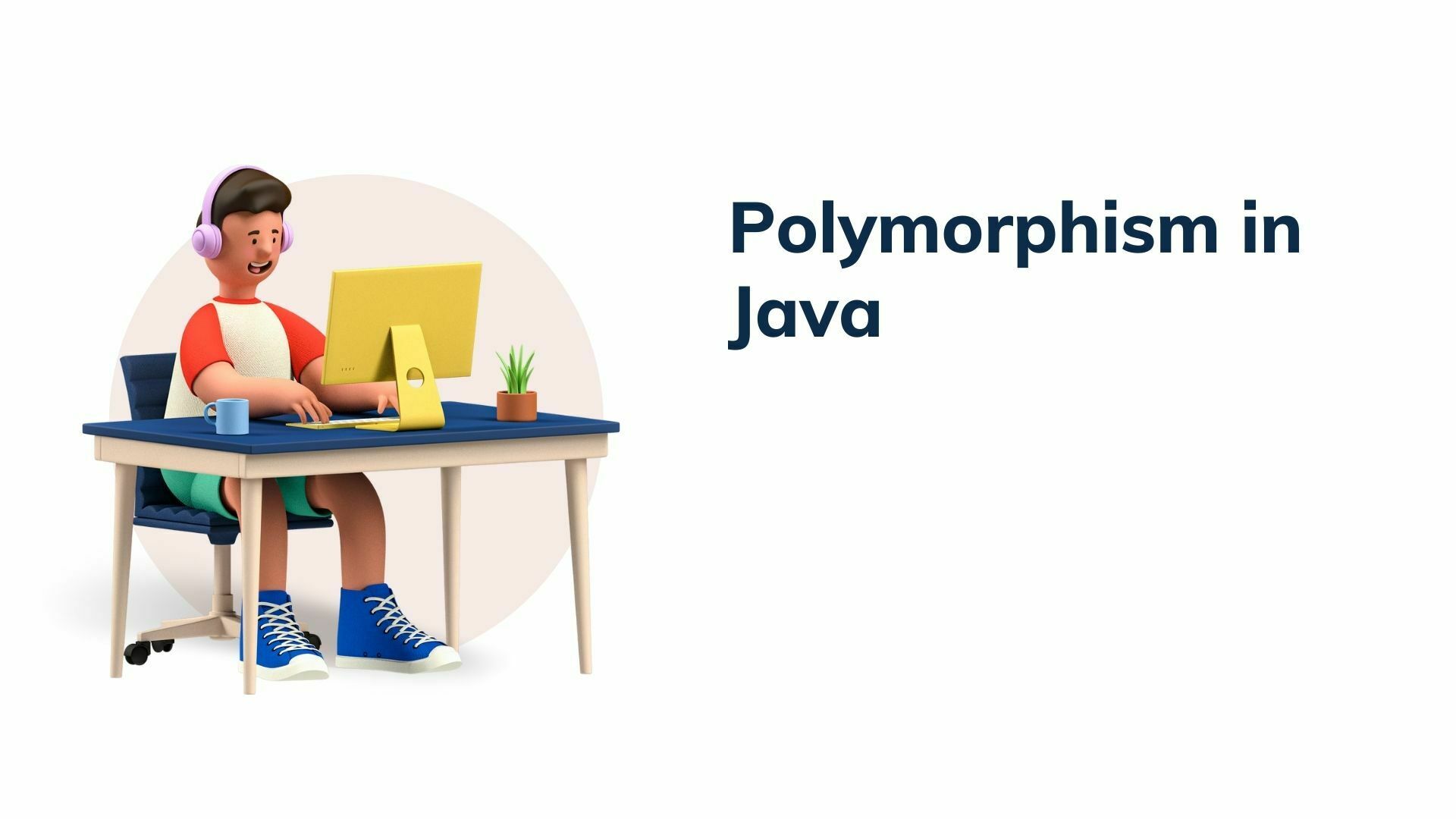
Polymorphism in Java is another important feature of the concept of OOP. We saw an overview of polymorphism in the tutorial OOP concepts in Java. In this tutorial, we will understand in detail polymorphism and its different types. We will cover about StaticPolymorphism, Dynamic Polymorphism, Execution Polymorphism.
What is polymorphism in Java
Polymorphism in Java as the name suggests means the ability to take multiple documents. It is derived from the Greek words where Poly means a lot and morph means forms. In Java, polymorphism in java is the same method that can be implemented in different ways. To understand this, we need to have an idea of inheritance in java as well as what we learned in the previous tutorial.
Types of polymorphism to Java
Below are the different types of polymorphism in java.
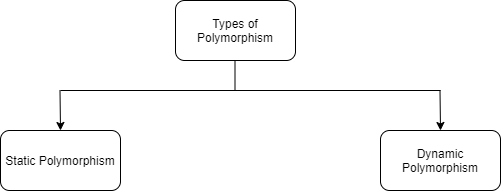
Static polymorphism
When we overchargon a static method, we call it static polymorphism. Since it solves polymorphism at compile time, we can also call it name as it does at polymorphism compilation. We can implement this type of polymorphism in java using either method overload or operator overload. In static polymorphism, at compile time, it identifies the method to call based on the parameters we pass.
Let’s understand this in detail with the help of examples.
Method Overloading
When there are many methods with the same name but different implementations, we call it a method overload. We can implement method overloading in two different ways:
- Different number of parameters
- Different types of parameters.
First, let’s look at an example of method overloading with a different number of parameters. In this example, we created a Region class that has 2 computeArea (int) and computeArea (int, int) methods. The difference between the two methods is that one has 1 parameter and the other has 2 parameters even though the method name is the same. So when we call the method using 1 parameter, it calls computeArea(int) and when we call the method with 2 parameters, it calls computeArea(int, int).

class Area{ public void computeArea(int a){ int area = a *a;System. out. println(“Area of square: ” + area); } public void computeArea(int a, int b){ int area = a*b;System. out. println(“Area of rectangle: ” + area); }}public class CalculateArea{ public static void main(String[] args){Area ar = new Area();ar. computeArea(5);ar. computeArea(4, 2); }}Area of square: 25Area of rectangle: 8
The following is an example of a method overload where the same method can have different types of parameters. In the code below, we have a subtract method with 2 different types of parameters. One has parameters of type int and the other has parameters of type double. Now, when we call the method using the class object, depending on the data type, it calls the corresponding method. Therefore, the first method calls subtract (int, int) since we pass integers. The second method calls subtract (double, double) since we pass double numbers.
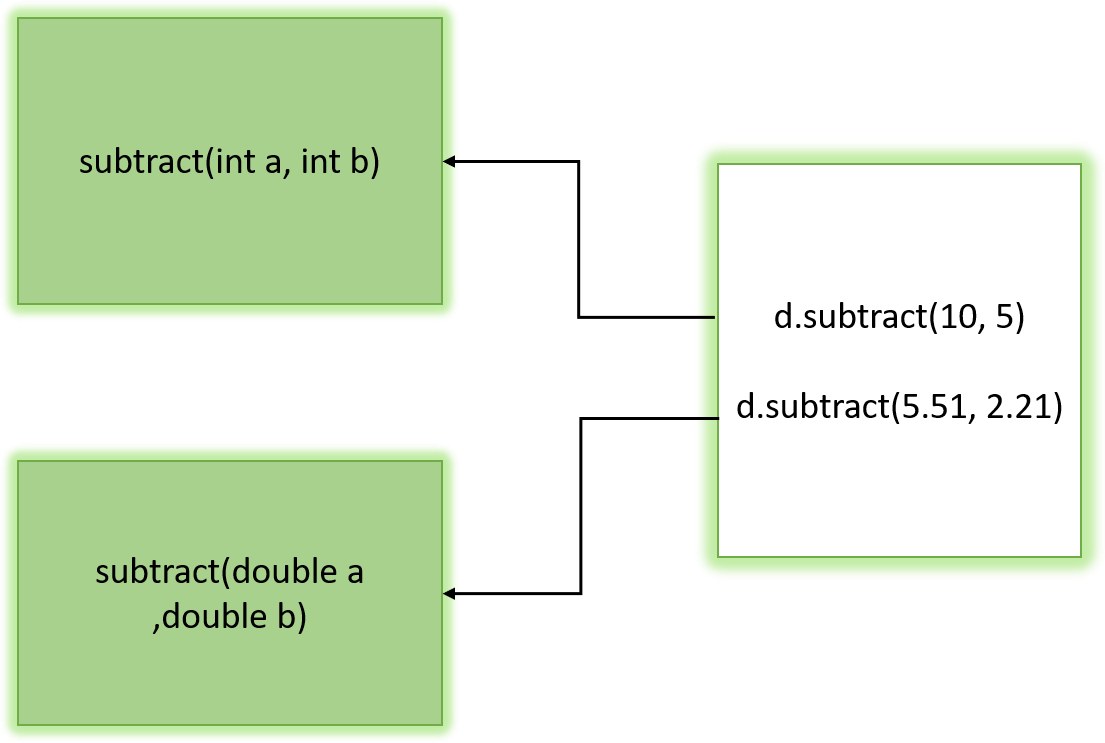
class Difference{ public void subtract(int a, int b){ int diff = a – b;System. out. println(“Difference: ” + diff); } public void subtract(double a, double b){ double diff = a – b;System. out. println(“Difference: ” + diff); }}public class MethodOverloadingDemo{ public static void main(String[] args){Difference d = new Difference();d. subtract(10, 5);d. subtract(5.51, 2.21); }}Difference: 5Difference: 3.3
Overloading the Operator
Similar to method overloading, we can also override an operator that is also an example of static polymorphism in java. But we can only overload the “+” in Java and does not support overloading other operators. When we use String as operands between + it results in Chain chaining. When we use numbers as operands between + it results in the addition of two numbers. The following is an example of + overloading the operator.class Operatordemo{ public void operator(String s1, String s2){ String s = s1+s2;System. out. println(“String concatenation: ” + s); } public void operator(int x, int y){ int sum = x + y;System. out. println(“Sum: “+sum); }}public class OperatorOverloading{ public static void main(String[] args){Operatordemo o = new Operatordemo();o. operator(“Good”, “Evening”);o. operator(4, 9); }}String concatenation: GoodEveningSum: 13
Dynamic polymorphism
When the polymorphism resolves during execution, we call it dynamic or execution polymorphism. In this type of polymorphism in java, it uses the reference variable of the superclass to call the overloaded method. This means that, depending on the object referenced by the reference variable, it calls the overloaded method of that corresponding class. We can use concept method replacement to implement dynamic polymorphism.
Method Replacement
When the subclass has the same method as the base class, we call it an overriding method, which means that the subclass has replaced the base class’s method. Depending on the type of object we create, it calls the method of that corresponding class. This means that if we create an object of the superclass and refer to it using the subclass, then it calls the method of the subclass. Since it computes this during execution, we call it as an execution polymorphism in java.
You will be able to clearly understand this concept with the example below. We have created a parent vehicle class and 2 subclasses Bike and car. The parent class has a speed method, and both subclasses have replaced the base class method with speed.
If we create an object of the instance Vehicle class and call the speed method (v.speed), it calls the method of the parent class. When we call the speed method using a created object of the instance Bike class (b.speed), it calls the method of the Bike class. Similarly, when we call the method using the created object of the Car instance class (c.speed), it calls the method of the Car class.

class Vehicle{ public void speed(){System. out. println(“Default speed”); }}class Bike extends Vehicle{ public void speed(){System. out. println(“Bike speed”); }}class Car extends Vehicle{ public void speed(){System. out. println(“Car speed”); }}public class VehicleType{ public static void main(String[] args){ Create an instance of VehicleVehicle v = new Vehicle();v. speed(); Create an instance of BikeVehicle b = new Bike();b. speed(); Create an instance of CarVehicle c = new Car();v. speed(); }}Default speedBike speedCar speed
Below is another example of a run-time polymorphism where we can use the same object name to create multiple class instances. We first declare an object f of fruit class and then using the Nine (ve) keyword we instantiate the object variable for the Fruit class. When we use this object to call the taste method, it calls the taste method of the Fruits class. Then, when we instantiate the object variable for the Apple class and call the taste method using that object, it calls the method of the Apple class. Similarly, this happens for the Pineapple class.Class Fruit{ public void taste(){System. out. println(“Fruits taste”); }}class Apple extends Fruits{ public void taste(){System. out. println(“Sweet taste”); }}class Pineapple extends Fruits{ public void taste(){System. out. println(“Sour taste”); }}public class FruitsDemo{ public static void main(String[] args){ Fruits f;f = new Fruits();f. taste();f = new Apple();f. taste();f = new Pineapple();f. taste(); }}Fruit taste” Sweet Taste “” Sour Taste “
Consider another example below where we call the method of a subclass that does not have a method overridden. In such a case, it calls the parent class method. The AnimalDemo class does not have the animalmethod. Therefore, it calls the Cow method because the AnimalDemo class extends the Cow class.Animal class { public void animaltype(){System. out. println(“Animal”); }}class Cow extends Animal{ public void animaltype(){System. out. println(“Herbivorous”); }}public class AnimalDemo extends Cow{ public static void main(String[] args){ Animal a;a = new AnimalDemo();a. animaltype(); }}Herbivorous
Executing polymorphism with data member
In run-time polymorphism, only the method is overridden and not the data member or variable. From the example below, we can clearly understand this difference. When we create an object b of the store class instance SBI class, it calls the superclass variable because the data members are not overwritten. In order to access the subclass variable, we need to create the object from the subclass ie SBI s = new SBI().Class Bank{ public double interestRate = 7.5;}class SBI extends Bank{ public double interestRate = 6.4;}public class BankDemo{ public static void main(String[] args){Bank b = new SBI();System. out. println(b.interestRate);SBI s = new SBI();System. out. println(s.interestRate); }}7.56.4
Difference Between Method Overloading and Method Override
Method Overloading | Method Replacement |
---|---|
This implements static polymorphism | This implements dynamic polymorphism |
This happens during compilation | This occurs during execution |
The same methods are present in the same class | The same methods are present in different classes |